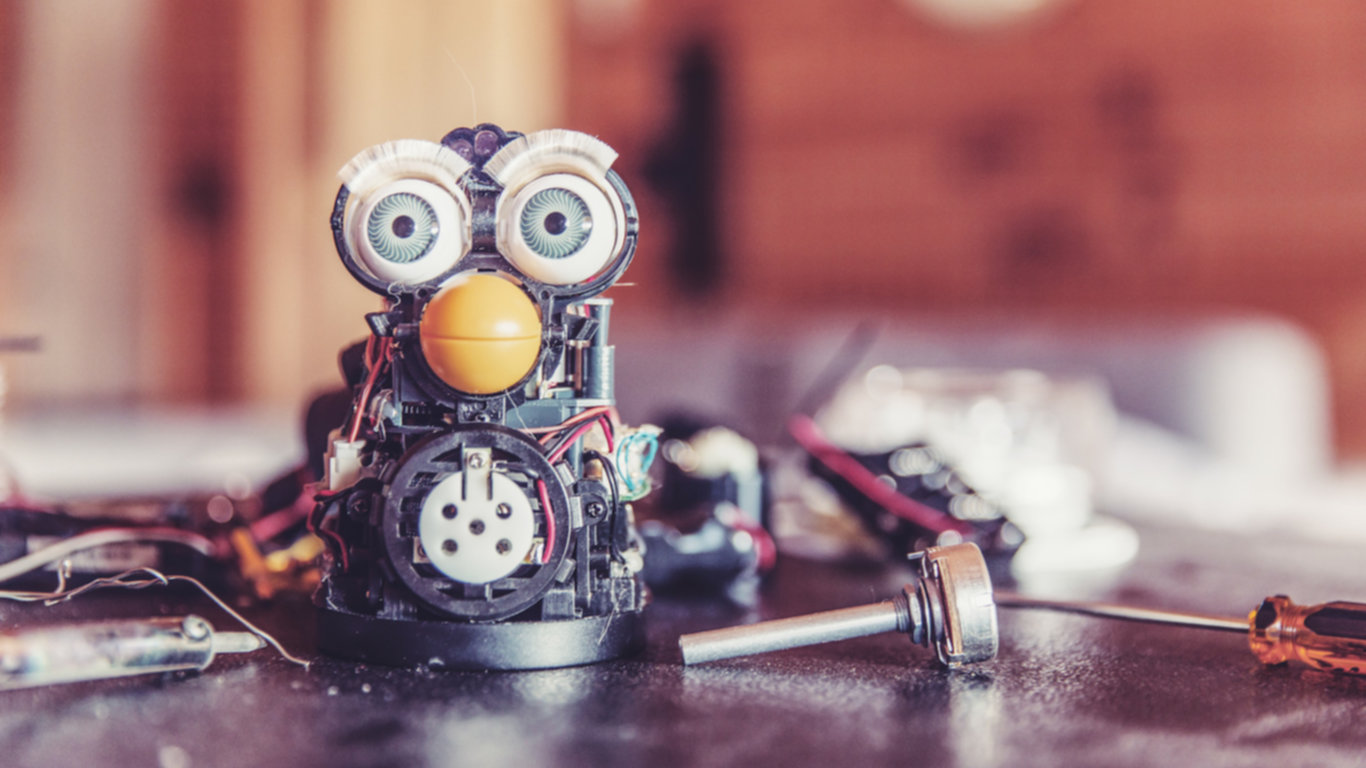
Testing is the only way to ensure that your code works.
That’s it, I said it. It’s the only thing you need to remember from this post. If you’re working with code, you are already somehow testing, right?
Maybe you do it by hand, you know, manually checking that the feature you just added works correctly. That’s a good start!
The problem with manual testing
The problem is that manual testing doesn’t scale. Let’s say you have an automated email being sent out anytime a user signs up. Are you doing it by hand? Of course not, because it doesn’t scale. If you have one new user per day, it might be fine (still, it would be a pain, let’s be honest).
When you have 10, 100 or even 100,000 new users every single day, you clearly cannot handle it manually anymore, so you just write code to deal with it. Now, let’s go back to testing. The example we just saw is a bit extreme when we’re talking about testing, I’ll admit it. Still, testing one feature is fine. Once you’ve added 20, 30 or more features to your application, are you still going to test them all when you change a piece of code?
Your answer is probably no. Personally, I wouldn’t do it (and I didn’t back in the days) because, well, it would take forever. I’d just test the latest feature I implemented, and maybe one that’s related. But I wouldn’t realize that X broke in the Y feature. Damn. Quick deploy on a Friday afternoon for a quick adrenaline rush, and off for a weekend at the beach. What I wouldn’t realize is the broken checkout process - a colossal loss of $1 billion over the weekend.
Scary story, huh?
It’s actually inspired from a real story, but the numbers have been inflated. A lot. Else they would have fired me!
Manual testing has its limits - our limits.
Everything is getting automated in our lives, why not automate the testing of our applications? You know, the applications that we created to automate something else that was too annoying to do manually.
Enter automated testing. It’s nothing new. Been around since people started writing code.
Wait… I don’t know what is “automated testing”!
Alright. Let’s me give you a quick explanation. The idea behind automated testing is to write a piece of code that only has one responsibility: ensure that a specific functionality in your application works as expected.
Automated testing includes the process of writing unit tests and integration tests. While unit tests are meant to test only “one unit” (a single component), integration tests are used to test multiple units, and how they interact together.
Now, let’s take a look at some concrete examples. Say we want to test the #add
method in the Calculator
class below.
# calculator.rb
class Calculator
def add(a, b)
a + b
end
end
And here is the method we would use to test that the #add
method works correctly. We first setup an instance of the Calculator
class, before using asset_equal
(provided by Minitest), to check that calling calculator.add(2, 4)
returns 6
as expected.
def test_add
calculator = Calculator.new
assert_equal 6, calculator.add(2, 4)
end
Here is the complete Minitest example, for reference.
require "minitest/autorun"
require "calculator"
class TestCalculator < Minitest::Test
def test_add
calculator = Calculator.new
assert_equal 6, calculator.add(2, 4)
end
end
Now that you have an idea of what a test looks like, let’s talk about why you would want to write them exactly.
Why write tests?
Spending time writing automated tests may seem counter-productive when you’re trying to push features out as fast as possible. What you need to realize is that automated tests are an incredible useful tool that have a huge range of benefits:
- Save time & money
- Once you’re good enough, you can actually develop faster by reducing the time you spend interacting with a web browser.
- The tests provide instant feedback on your code, allowing quick refactoring, forcing you to write code in a smarter way and reducing the coupling between your components.
- Tests will allow you to catch bugs early on, before pushing anything to production.
- A good set of tests for an application makes it super easy to introduce new comers to the codebase. They can play around, try to fix bugs or add features and the test suite will tell them if they broke anything.
- Tests give you confidence in your code.
- A test suite is an always up-to-date documentation for your code. If someone wants to know how a piece of code works, they can just checkout the logic in the tests.
Even if these don’t sound good enough for you, wait until you try it to make a judgement ;)
Going further
Learning how to write tests takes longer than reading an article on the topic. If you’re really serious about making your code better, faster and stronger, you might want to checkout this course I’m building: Rails 5 Testing - Why, Where and How?,