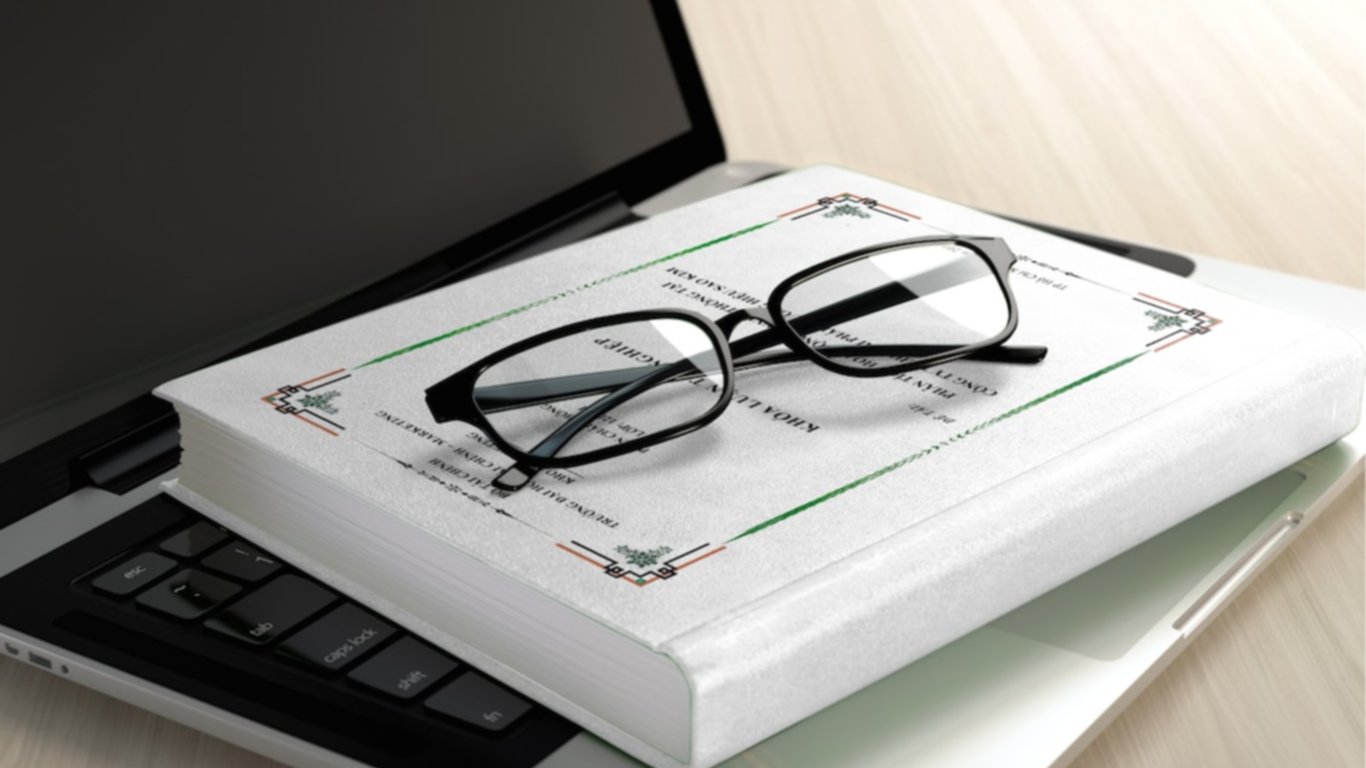
UPDATE: I’ve since then wrote and published a book on how to build Web APIs with Ruby.
This article is a very basic introduction to web APIs and how to build them with Ruby.
What is an API
As you probably know, API stands for Application Programming Interface.
Let’s checkout the definition from Wikipedia.
In computer programming, an application programming interface (API) is a set of routines, protocols, and tools for building software applications. An API expresses a software component in terms of its operations, inputs, outputs, and underlying types.
Basically, you’re building stuff so you or other people can build more stuff on top of it.
In the case of a programming library, the API is the way you interact with it. It’s the ‘interface’ that defines how you ask for something and how you set something. Some APIs are beautiful, others are not. That usually means they are either well-conceived, easy to use and properly documented or the complete opposite: hard to understand and cumbersome.
What is a Web API
A web API follows the same idea. Extend the concept we defined for a library to a web application. You’re basically building something for other people to consume in mobile applications, javascript applications or other web applications. The main difference is that the communication is happening through HTTP (mostly).
A lot of known platforms provide APIs to integrate with them. For example, Facebook offers ways to query for a user information or his friends by using the right security details.
Understand the difference between web APIs and web services
You know I like to use Wikipedia, so here are the entries for those two terms.
Web services
The W3C defines a Web service generally as a software system designed to support interoperable machine-to-machine interaction over a network.
The W3C Web Services Architecture Working Group defined a Web Services Architecture, requiring a specific implementation of a “Web service.” A Web service has an interface described in a machine-processable format (specifically WSDL). Other systems interact with the Web service in a manner prescribed by its description using SOAP (Simple Object Access Protocol) messages, typically conveyed using HTTP with an XML serialization in conjunction with other Web-related standards.
Web API
A server-side web API is a programmatic interface to a defined request-response message system, typically expressed in JSON or XML, which is exposed via the web—most commonly by means of an HTTP-based web server.
The Difference
Basically, web services are a standard defined by W3C with a specific set of rules. Web services are often known to rely on WSDL, SOAP and XML.
Web APIs are much simpler. Just do it the way you want it. There are no standards. However web APIs usually use the HTTP protocol to communicate, can follow the REST style and exchange data with XML or JSON. Again, there are no standards but it’s been proven to be a great way to build a web API in the past years.
Why build a Web API
A lot of scenarios can require a web API. Here are a few examples:
- Persistence for mobile applications
Since mobile applications are run inside sandboxes and cannot access durable persistence, it is often required to build a web APIs to do this for them. Saving a user’s details on the server side gives a better user experience by allowing the use of multiple devices and no data loss when a phone is reset or broken.
- Mobile application with interactions between users
Another use case for a web APIs would be to create interactions between users. Like in a social media or a dating application. To be able to match all those people, you need them in the same place and that’s not going to be inside a smartphone. That’s where a web API comes into play.
- Mobile application for an existing web platform
This one is quite obvious. If you currently have a web platform, like Facebook for example, and you wish to have the same features inside a mobile application, you will need to add a web API to your platform.
- Javascript SPA
Singe page applications built with javascript are always meant to work with a backend.
Why use Ruby to build a web API
Ruby is a great programming language. It might not be the fastest kid around but it’s still a great choice to build a web API. Not only it’s great to code in Ruby, there is also a lot of great tools to build web APIs specifically. From testing to routing, everything is covered and you will be able to pick the right tool for your needs.
There are other options though. Python and Node.js are too other good choices. If you prefer a compiled language, C# and the .net framework comes with a lot of nice utilities designed to create web APIs (ASP.NET for example).
I will mainly be talking about Ruby APIs in this article and the following ones because that’s simply my favorite tool.
Available tools/frameworks
As I said earlier, there are a lot of tools to build web APIs with Ruby. In this section, I’ll talk about a few frameworks available.
Grape
Grape is a micro-framework providing a way to build lighweight REST-like API by using a simple DSL. If you don’t need a heavy framework like Rails, Grape could be the best choice for you!
Here’s a small code example from GitHub:
module Twitter
class API < Grape::API
version 'v1', using: :header, vendor: 'twitter'
format :json
prefix :api
helpers do
def current_user
@current_user ||= User.authorize!(env)
end
def authenticate!
error!('401 Unauthorized', 401) unless current_user
end
end
resource :statuses do
desc "Return a status."
params do
requires :id, type: Integer, desc: "Status id."
end
route_param :id do
get do
Status.find(params[:id])
end
end
end
end
end
Checkout Grape!
Sinatra
Sinatra also offers a simple DSL to quickly build web APIs in Ruby without much effort. Here’s the hello world
example found on Sinatra website:
# myapp.rb
require 'sinatra'
get '/' do
'Hello world!'
end
Checkout Sinatra!
Rails-API
Rails-API is a slimmed up Ruby on Rails meant to specifically create web APIs. By removing uninteresting modules (for an API), Rails-API allows you to build faster applications.
Checkout Rails-API!
Rails
Finally, you can just use Ruby on Rails to build a web API. Don’t think it’s overkill just because you’re not dealing with HTML. You still need to implement all the logic somewhere and Rails conventions will help you keep stuff organized and clean. However, there are a few things you can do to not use things included in Rails that you don’t need. For example, consider inherit from ActionController::Metal
(doc) and only load the modules that you need.
Checkout Rails!
Conclusion
That’s it for this article. This was meant as a general introduction to building web APIs with Ruby. In the next article, we’ll dive into REST and what it means to be RESTful!
I’ve just started to write a book about building web APIs using Ruby. You can read more about it and get the latest updates here.