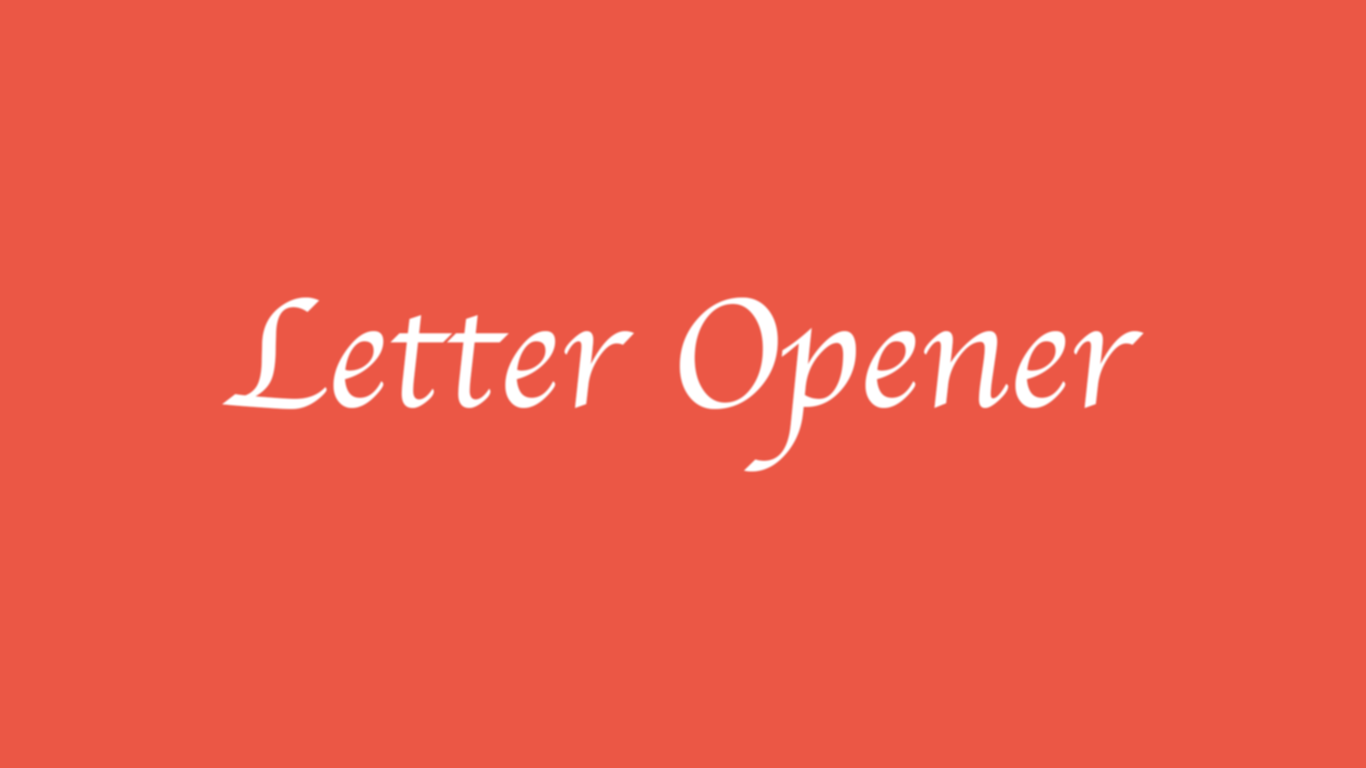
Checking that emails are correctly sent on your development machine can be hard since you often don’t have a functional SMTP server and you don’t want to send emails everywhere anyway. How to ensure that your emails are correctly sent and at the right time? Letter Opener is here for you.
Letter Opener is a nice little gem created by Ryan Bates that will allow you to preview sent emails in your browser. Let’s see how it works!
The Project
To learn how to use Letter Opener, we’re going to create a super simple Rails application. We’ll install the gem, configure it and then create an email that will be sent every time we access the main page of our application.
Versions used
For this jutsu, I used the following version of Ruby and Ruby on Rails.
Ruby: 2.2.0
Ruby on Rails: 4.2.0
1. Generate a Rails application
First, let’s create a new application. Navigate to your projects folder and run the following command.
rails new letter_opener_jutsu
2. Install Letter Opener
Then we need the Letter Opener gem. Since we’re only going to use it in development, we can specify the development
group.
gem "letter_opener", :group => :development
Don’t forget to bundle.
bundle install
3. Config Letter Opener
We need to tell our Rails application to use Letter Opener in development. To do this, we can simply add the following line to the development environment file: development.rb
.
# letter_opener_jutsu/config/environments/development.rb
config.action_mailer.delivery_method = :letter_opener
We can now start our server and set up some basic scaffolding before sending some test emails.
rails server
4. Create a basic view for root
We’re going to scaffold user
in order to have some basic views. We won’t do much on those views.
Let’s run the scaffold generator.
rails g scaffold user email:string
Migrate the generated migration.
rake db:migrate
Set the root of our application to the index action on the users controller.
Rails.application.routes.draw do
resources :users
root 'users#index'
end
Access http://localhost:3000
and you should see the index view for users. Everything looks fine!
5. Create a mailer
Now we can create a mailer. We’re going to use a Rails generator to do that quickly.
rails g mailer UserMailer
Let’s add the method that will be responsible for our little example email to the UserMailer
.
# letter_opener_jutsu/mailers/user_mailer.rb
class UserMailer < ApplicationMailer
def example(user)
@user = user
mail(to: @user.email, subject: 'Test Email for Letter Opener')
end
end
And the corresponding view: letter_opener_jutsu/app/views/user_mailer/example.html.erb
<!-- letter_opener_jutsu/app/views/user_mailer/example.html.erb -->
<h1>Welcome <%= @user.email %>! </h1>
Finally, we need to actually send the email. Let’s put the code to do this in the index
action of the UsersController
. Don’t do that in a real application, that would be quite annoying ;)
# letter_opener_jutsu/app/controllers/users_controller.rb
# ...
def index
UserMailer.example(User.new(email: 'bo@samurails.com')).deliver
@users = User.all
end
# ...
6. Preview it!
Access http://localhost:3000
. A new tab should be opened in your browser showing the email that we just created.
[
7. Work on your email
Letter Opener is great to see when emails are actually sent and if everything looks correct inside each email. However, when desiging an email, there are better and faster ways to preview your email.
When we generated our mailer, Rails also created a file named user_mailer_preview
in the test folder. In this file, we can create one method for each of our emails to preview them super easily in the browser.
This is actually a feature from the mail_view gem that was integrated in Rails 4.1. If you use an older version of Rails, you can simply add the gem to your application.
Let’s add the method for our example
email:
# letter_opener_jutsu/test/mailers/previews/user_mailer_preview.rb
# Preview all emails at http://localhost:3000/rails/mailers/user_mailer
class UserMailerPreview < ActionMailer::Preview
def example
UserMailer.example(User.new(email: 'bo@samurails.com'))
end
end
Now we can head to the url http://localhost:3000/rails/mailers/user_mailer
to see a list of available emails to test.
[
After clicking on example
, we can preview the email we are currently working on. If you make any change to example.html.erb
, you can simply refresh this page and see the changes.
[
The Source Code
The Source Code is available on Github.
The End
That’s it for this jutsu about Letter Opener. I hope you learned a few things on the way! If not, let me know what could be added to this jutsu to make it better.