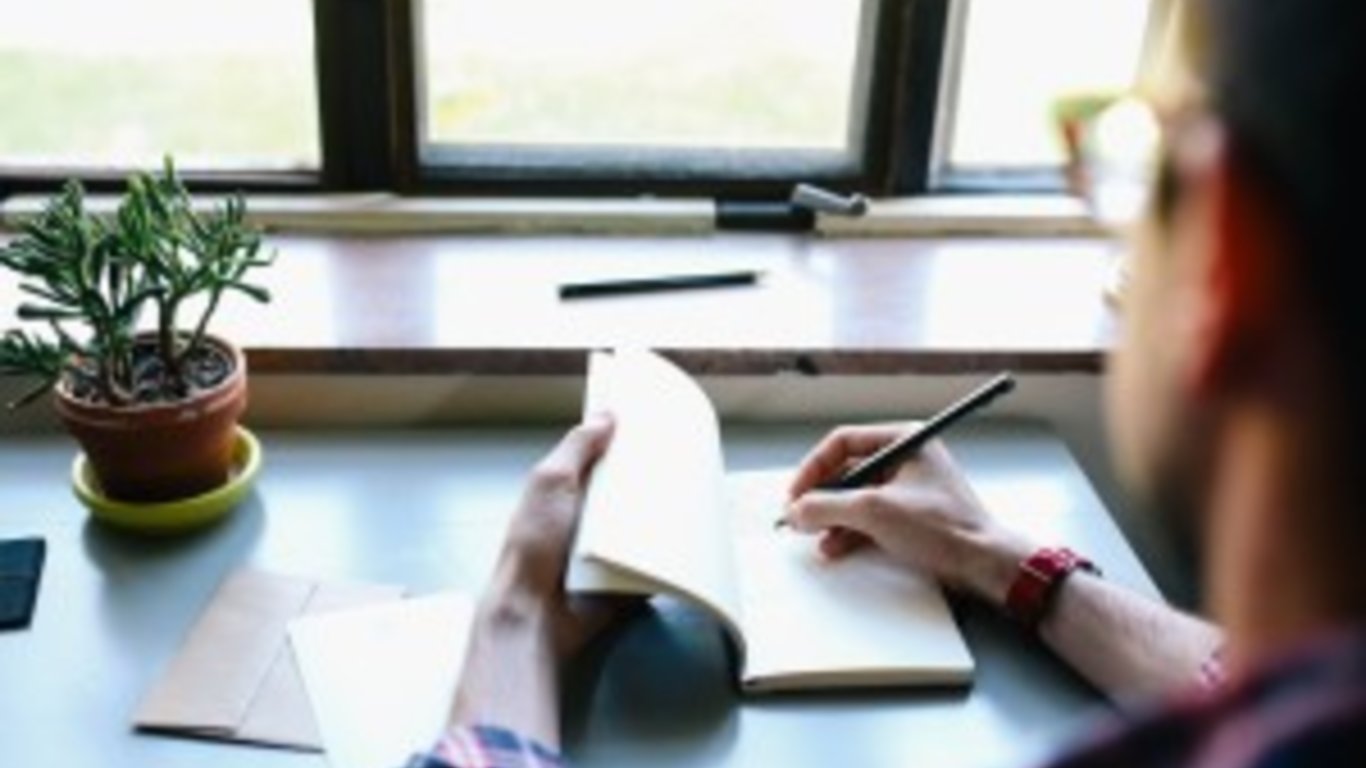
This guide is the first part of a series, Prepare for a Ruby job interview, that will teach you (or help you remember) some basic stuff that you should know as a Ruby developer.
Prepare for a Ruby job interview
The full list of articles is available below.
- Prepare for a Ruby job interview
- Ruby Inheritance, Encapsulation and Polymorphism
- Ruby & Duck Typing
- Include vs Extend in Ruby
- Overview of loops in Ruby
- Ruby Blocks : Procs and Lambdas
- Ruby & the Splat Operator
- Random Ruby Tricks & Info
- Ruby FizzBuzz & Fibonacci
- Big O Notation & Complexity in Ruby
Programming Concepts
For this first lesson, we’ll go over basic principles of programming.
Let’s start with a few definitions.
Class
Classes are the blueprint from which objects are created. They hold data and have methods that interacts with that data.
Object
An object is an instance of a class. Everything in Ruby is an object (Even classes which descend from the Object root class!).
A bit more about this root class :
Object is the default root of all Ruby objects. Methods on object are available to all classes unless explicitly overridden.
Atually Object inherit from BasicObject, a blank class :
BasicObject is the parent class of all classes in Ruby. It’s an explicit blank class.
BasicObject can be used for creating object hierarchies independent of Ruby’s object hierarchy, proxy objects like the Delegator class, or other uses where namespace pollution from Ruby’s methods and classes must be avoided.
Module
A Module is a collection of methods and constants. The methods in a module may be instance methods or module methods. Instance methods appear as methods in a class when the module is included, module methods do not. Conversely, module methods may be called without creating an encapsulating object, while instance methods may not.
Modules can be used as a namespace mechanism. They cannot be instanciated, as opposed to classes. They can be used as mix-ins to add logic to a class (Multiple Inheritance)
Method vs Function
Many languages distinguish between functions, which have no associated object, and methods, which are invoked on a receiver object.
In Ruby, all methods are true methods and are associated with at least one object. If you declare a method without being inside a class, the method will automatically be added to the Object class.
The only functions you will find in Ruby are proc and lambas that we’ll see later.
Level of accessibility
There are 3 levels of accessibility for a class method in Ruby :
- Public : Can be called from outside the class.
- Protected : Can be called from outside only by the defining class or its subclasses.
- Private Can be called only from inside the defining class by the definind class or its subclasses.
Class method vs instance method
A class method is a method defined on a class. You can define one like this :
class Document
self.do_something
end
end
or
class Document
class << self
def do_something
end
end
end
And you can call it like this :
Document.do_something
An instance method is a method available on an instance of a class. You probably already know how to create one :
class Document
def do_something
end
end
And you need to instantiate an object before you can call it :
Document.new.do_something
Class variable vs instance variable vs module variables
Class Variables are defined with @@
. A Class variable is shared between all instances and children.
class Document
@@stuff = []
def self.stuff
@@stuff
end
def stuff
@@stuff
end
end
class XmlDocument < Document
end
Document.stuff << 1
# => [1]
XmlDocument.stuff << 2
# => [1, 2]
Document.new.stuff << 3
# => [1, 2, 3]
Since classes are objects, you can define instance variable on a class with @
:
class Document
@stuff = []
def self.stuff
@stuff
end
def stuff
@stuff
end
end
class XmlDocument < Document
end
Document.stuff << 1
# => [1]
XmlDocument.stuff << 2
# => NoMethodError: undefined method `<<' for nil:NilClass
Document.new.stuff << 2
# => NoMethodError: undefined method `<<' for nil:NilClass
Just like instance variables for classes, instance variables are defined with @
on regular instances :
class Document
def initialize
@stuff = rand(10)
end
def stuff
@stuff
end
end
Document.stuff
# => NoMethodError: undefined method `stuff' for Document:Class
Document.new.stuff
# => 5
Document.new.stuff
# => 1
That’s it for the first part. Checkout the next article about Inheritance, Encapsulation and Polymorphism.