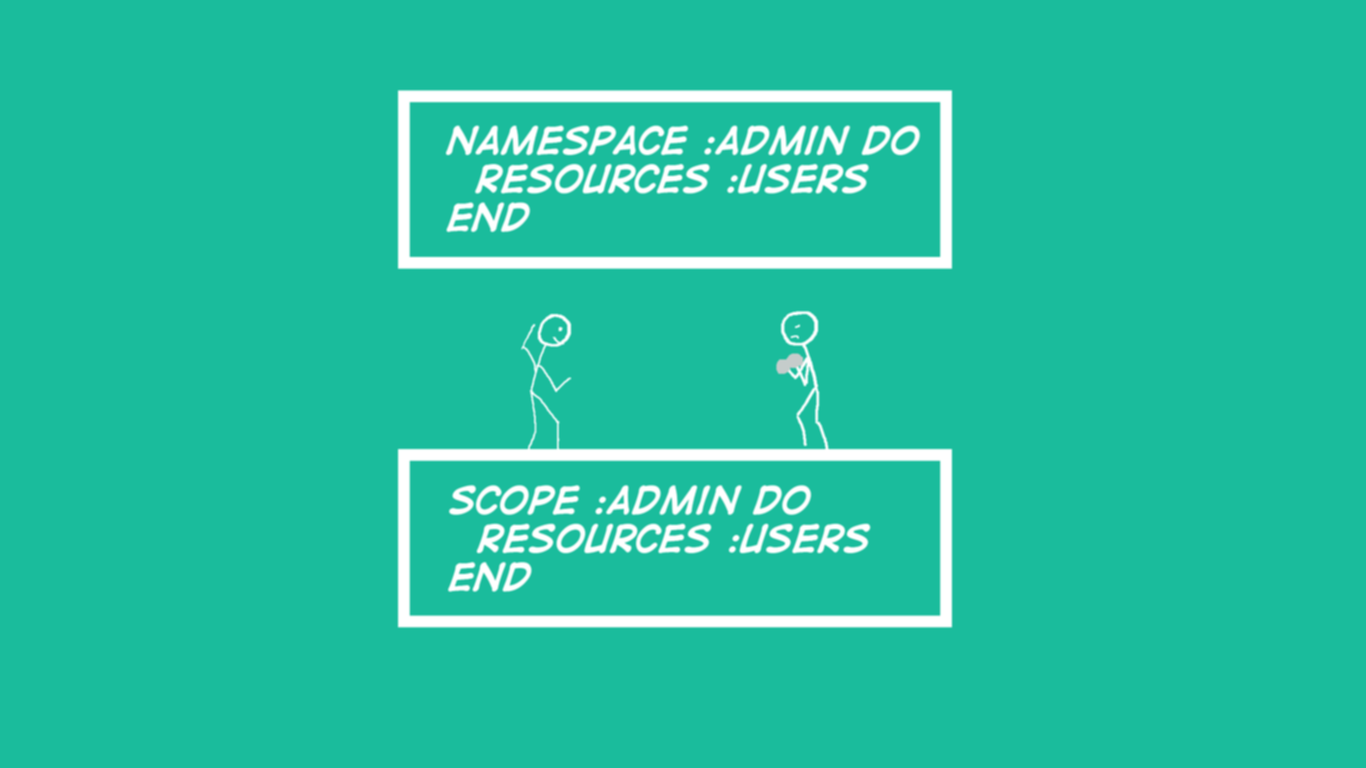
You may be wondering about the difference between scope
and namespace
for the routes in your Rails application. It’s important to know the difference, because they affect the path of your resources and the controllers.
namespace
This is the simple option. When you use namespace
, it will prefix the URL path for the specified resources, and try to locate the controller under a module named in the same manner as the namespace.
With the following code in the routes.rb
file,
namespace :admin do
resources :users
end
You will end up with the following routes:
Prefix Verb URI Pattern Controller#Action
admin_users GET /admin/users(.:format) admin/users#index
POST /admin/users(.:format) admin/users#create
admin_user GET /admin/users/:id(.:format) admin/users#show
PATCH /admin/users/:id(.:format) admin/users#update
PUT /admin/users/:id(.:format) admin/users#update
DELETE /admin/users/:id(.:format) admin/users#destroy
As you can see, admin
was added as a prefix in the URI path (/admin/users
) and as a module containing the controller (admin/users#index
). With this code, Rails will expect Admin::UsersController
to be located at app/controllers/admin/users_controller.rb
.
scope
scope
is a bit more complex - but the advantage is that it gives you more options to fine-tune exactly what you want to do.
No Options
When using scope
without any options and only a scope name, it will just change the resources path.
# routes.rb
scope :admin do
resources :users
end
Here are the generated routes:
Prefix Verb URI Pattern Controller#Action
users GET /admin/users(.:format) users#index
POST /admin/users(.:format) users#create
user GET /admin/users/:id(.:format) users#show
PATCH /admin/users/:id(.:format) users#update
PUT /admin/users/:id(.:format) users#update
DELETE /admin/users/:id(.:format) users#destroy
As you can see, /admin
was added as a prefix before /users
, but the users
controller doesn’t have to be inside any module.
With Options
scope
supports three options: module
, path
and as
.
module
module
lets us define in which module the controller for the embedded resources will live.
scope module: 'admin' do
resources :users
end
Prefix Verb URI Pattern Controller#Action
users GET /users(.:format) admin/users#index
POST /users(.:format) admin/users#create
user GET /users/:id(.:format) admin/users#show
PATCH /users/:id(.:format) admin/users#update
PUT /users/:id(.:format) admin/users#update
DELETE /users/:id(.:format) admin/users#destroy
path
path
allows us to set the prefix that will appear in the URI, before the resource name.
scope module: 'admin', path: 'fu' do
resources :users
end
Prefix Verb URI Pattern Controller#Action
users GET /fu/users(.:format) admin/users#index
POST /fu/users(.:format) admin/users#create
user GET /fu/users/:id(.:format) admin/users#show
PATCH /fu/users/:id(.:format) admin/users#update
PUT /fu/users/:id(.:format) admin/users#update
DELETE /fu/users/:id(.:format) admin/users#destroy
as
Finally, as
can be used to change the name of the path method used to identify the resources.
scope module: 'admin', path: 'fu', as: 'cool' do
resources :users
end
In this case, we now have cool
as a prefix for the path names cool_users
and cool_user
.
Prefix Verb URI Pattern Controller#Action
cool_users GET /fu/users(.:format) admin/users#index
POST /fu/users(.:format) admin/users#create
cool_user GET /fu/users/:id(.:format) admin/users#show
PATCH /fu/users/:id(.:format) admin/users#update
PUT /fu/users/:id(.:format) admin/users#update
DELETE /fu/users/:id(.:format) admin/users#destroy