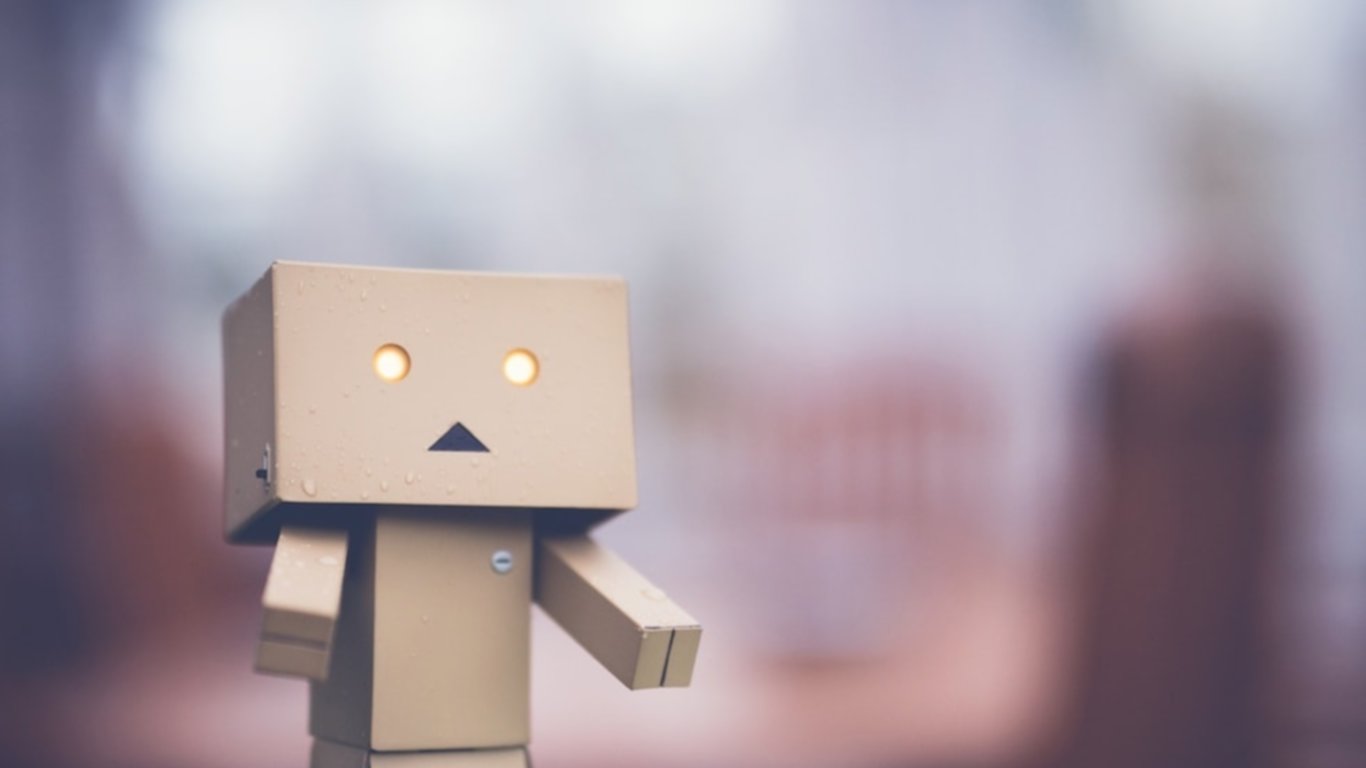
CAPTCHAs are an awesome way to protect your forms from automated submissions using Bots. The only problem? They suck.
CAPTCHA Systems suck!
Think about it. You’re trying hard to convert people and get them to signup on your website. Asking them to calculate the result of 2 plus 2 or recognize some characters is a great way to send people away. How many times have you submitted forms with a CAPTCHA to realize that you weren’t able to differentiate ‘b’ and ‘lo’ in a distorted image?
Google is here to the rescue
Fortunately, Google released a new CAPTCHA system that only requires people to click on a checkbox. Yes, that’s right, a simple click!
Wait, how does this work?
Honestly, I have no idea and Google is not going to tell us. It’s probably based on examining users’ behavior on past websites, analyzing cookies and so on. Anyway, it looks great and it works!
Here’s a quick screenshot of the CAPTCHA:
Now, it’s time to build stuff with it ;)
Let’s build something!
In this Railsjutsu, we’re going to build an application with a form to create messages. To avoid spammers and bots, we’ll integrate Google ReCaptcha.
1. Setup
Our application is going to be super simple. It will only contain one controller/model and the corresponding views.
Let’s quickly generate the application.
rails new recaptcha_jutsu
2. Create a Controller
Now that we have an application, we will scaffold a Message
entity. Run the following command to generate the model, the controller, the views and the tests.
rails g scaffold Message name:string email:string content:text
And don’t forget to run the migrations.
rake db:migrate
We also need to define the root path of our application. To do this, we have to change the routes.rb
file:
Rails.application.routes.draw do
resources :messages
root 'messages#new'
end
Now let’s run our server with rails server
. If you head over to http://localhost:3000
, you should be able to see the new message form. Feel free to create as many new messages as you want. We could actually create a bot to fill this form and submit it.
That’s exactly what we’re trying to prevent so let’s see how we can use Google ReCaptcha to prevent it.
3. Adding Google ReCaptcha
Google ReCatpcha is pretty easy to add on its own. All you need to do is go to Google’s website (here), click on ‘Get ReCaptcha’, register a website, copy the code into your application and that’s it!
But there is actually a simpler way for us, lucky Rails developers. Of course, there is a gem: recaptcha.
Let’s use it in our application.
4. Adding the recaptcha
gem
We first need to edit the gemfile
, add the following and then run bundle install
.
gem "recaptcha", require: "recaptcha/rails"
To work properly, we need to set the API Key provided by Google. You should be able to find it there if you don’t have it yet.
In your applications and in production, you should use some environment tool to handle secret keys but in this simple example, I’m just going to put it in an initializer.
Create a file named config/initializers/recaptcha.rb
and add the following inside:
Recaptcha.configure do |config|
config.public_key = 'YOUR_PUBLIC_KEY'
config.private_key = 'YOUR_PRIVATE_KEY'
end
Restart your application if it’s already running to get the configuration active.
5. Adding the CAPTCHA to the new message form
We just have one line to add in our view. Open the app/views/messages/_form.html.erb
file and add <%= recaptcha_tags %>
to it.
<!-- app/views/messages/_form.html.erb -->
<%= form_for(@message) do |f| %>
<!-- ... -->
<%= recaptcha_tags %>
<div class="actions">
<%= f.submit %>
</div>
<% end %>
If you reload the page you should now see the following:
That’s it for our form. Don’t forget to play with the CAPTCHA, everything should work fine.
6. Checking the CAPTCHA result in the controller
We need to change the create
action in the MessagesController
to check if the CAPTCHA was correctly validated. To do this, we just need to call the method named verify_recaptcha
provided by the recaptcha
gem.
# app/controllers/messages_controller.rb
def create
@message = Message.new(message_params)
respond_to do |format|
# We change the following line
if verify_recaptcha(model: @message) && @message.save
format.html { redirect_to @message, notice: 'Message was successfully created.' }
format.json { render :show, status: :created, location: @message }
else
format.html { render :new }
format.json { render json: @message.errors, status: :unprocessable_entity }
end
end
end
Now it’s time for you to play with it. Try submitting with and without the CAPTCHA. Now you can prevent bots from spamming your forms.
It’s amazing, isn’t it?
7. Changing the theme
ReCaptcha comes with a few settings you can change. For example, we can set the theme to dark to have something like this:
The code for this is just:
<%= recaptcha_tags theme: 'dark' %>
You can see all the options and all the documentation for ReCaptcha here.
Wrap Up
That’s it for this RailsJutsu. I hope you enjoyed learning how to use Google ReCatpcha. I personally love it and have been using on a few web applications already!